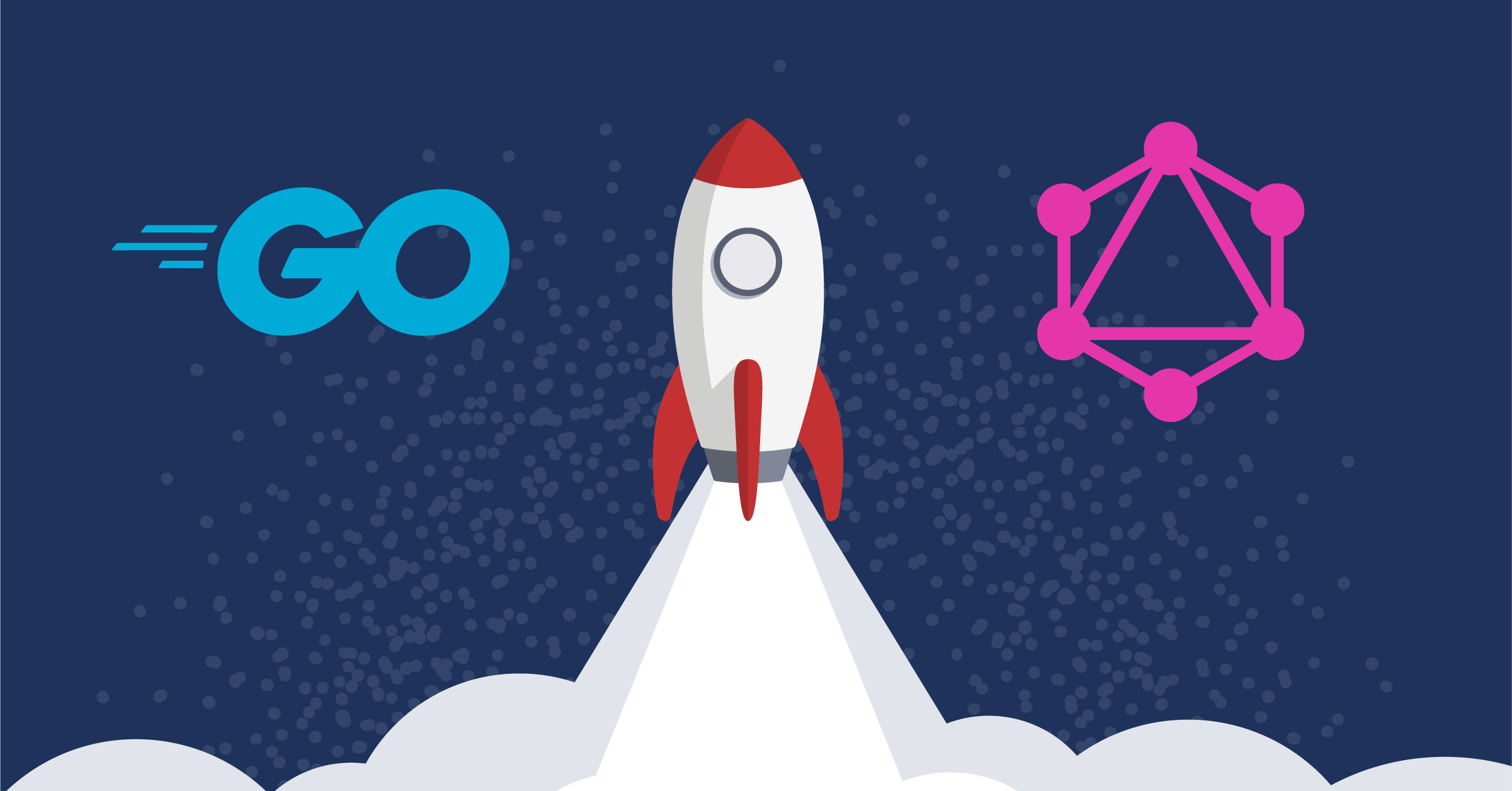
Building CRUD GraphQL APIs in Go
GraphQL APIs are gaining traction due to their ease of use, flexibility, and efficiency. GraphQL APIs are relatively easy to build, manage, and consume. GraphQL is a specification for building APIs, and anyone can write GraphQL APIs in any server-side programming language of choice. This tutorial teaches you how to build CRUD GraphQL APIs in Go using the gqlgen library.
There are many packages for building GraphQL APIs in Go; the most popular ones are the graphql-go and gqlgen libraries. The graphql-go package takes a code-first approach, so you won’t need to define a schema to build a GraphQL server as opposed to the gqlgen library, which takes a schema-first approach. Overall, the gqlgen library is more widely adopted by developers.
Getting started with the glqgen library
Prerequisites
You’ll need to meet these requirements to get the most out of this hands-on tutorial.
1. Experience working with Go, and a recent version of Go installed on your machine.
2. Knowledge of SQL and the GORMpackage is a bonus.
The gqlgen library provides many features and functionalities to help build GraphQL APIs in Go quickly. Start by defining your schema in the Schema Definition Language (SDL). Then the library would generate most of the code needed for the API.
You can find the code for this tutorial on my GitHub repository. Otherwise, follow the steps below to set up the environment yourself.
Initialize a new go module in your working directory
mkdir gqlgen-example
cd gqlgen-example
go mod init gqlgen-example
Run this command on the terminal in your working directory to install the gqlgen library in your Go workspace.
printf '// +build tools\npackage tools\nimport _ "github.com/99designs/gqlgen"' | gofmt > tools.go
go mod tidy
The command adds the gqlgen library to your Go modules and all the dependencies the project needs. After installing the gqlgen
package, run this command for the gqlgen package to generate the necessary files and configurations you’ll need for the GraphQL API.
go run github.com/99designs/gqlgen init
The command generates a graph
directory and many other files you’ll need for the API.
The code for running the GraphQL server is in the server.go
file. Run the file to start the server.
go run server.go
By default, the server runs on port 8080
. You can edit the server.go
file to edit the port number. Opening the port on your browser displays a GraphiQL user interface that you can use to interact with the GraphQL API and read the API documentation.
Defining a GraphQL schema using the GraphQL SDL
The gqlgen
package takes the schema-first approach, and the library needs a schema to generate the API code. In the graph
directory the package generated, you’ll find the schema.graphqls
file. Edit the schema based on your API.
Here’s the schema for the GraphQL API you’ll build in this tutorial.
type BioData {
name: String!
Age: Int!
}
input AddBio{
name: String!
Age: Int!
}
type Mutation{
CreateBio(input: AddBio!): BioData!
DeleteBio(id: Int!): String!
UpdateBio(id: Int!): String!
}
type Query {
GetBio(id: Int!): BioData!
GetBios: [BioData!]!
}
The GraphQL schema above has mutation (update, delete), input(post), and query(get) functionalities in the definition since those are the operations for this tutorial.
If you replace the file’s contents with the code above, you may need to remove CreateTodo and Todos from the end of schema.resolvers.go. After defining your GraphQL schema and cleaning your resolvers file, run this command in your workspace:
go run github.com/99designs/gqlgen generate
The gqlgen
package will regenerate the graph
package in your workspace based on your schema. Once gqlgen
regenerates the files, you can edit the files to get your GraphQL API and server running.
Setting up the GraphQL server
In the server.go
file; the main
function starts the GraphQL server on a specified port. Change the port or edit the file based on your preferences. The default port is 8080; run the main function or the server file to start the server. The GraphQL server could take a few minutes to load.
Setting up the SQL database with GORM
For this tutorial, we’re using a SQL database (SQLite) as a data store for the API. GORM is the most popular ORM in the Go ecosystem, and you’ll use it to auto-migrate and interact with the database.
Run this command to install the GORM package and the GORM SQLite driver in your workspace:
This blog post was created as part of the Mattermost Community Writing Program and is published under the CC BY-NC-SA 4.0 license. To learn more about the Mattermost Community Writing Program, check this out.