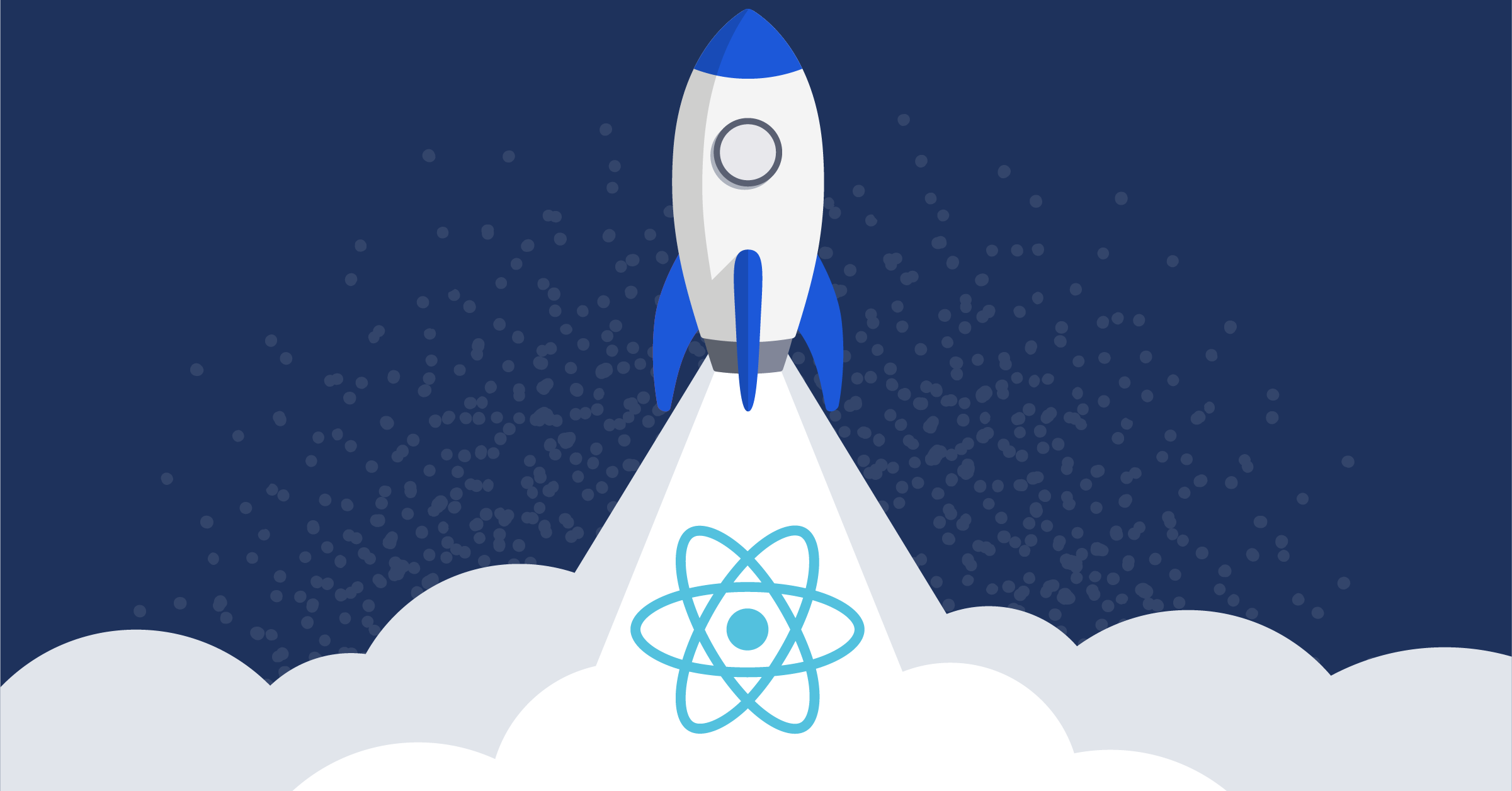
How to deploy a React app to Kubernetes using Docker
The concept of containerization helps you run applications as lightweight virtual machines. As a web developer, setting up local development environments can be tiresome. However, using tools like Docker and Kubernetes gives developers an upper hand to quickly set up and deploy applications.
This guide uses Docker to deploy a React app to Kubernetes.
- Prerequisites
- Setting up Minikube and Kubectl
- Setting up the React application
- Write the Dockerfile
- Running the React app on Nginx
- Running the React app on Docker
- Deploying the Docker image
- Learn more about building apps with Kubernetes
Prerequisites
Before you proceed with this tutorial, make sure you have the following tools installed on your computer:
Setting up Minikube and Kubectl
To correctly run these tools:
- First, ensure your installed Docker is up and running.
- Install Minikube using any of these options.
- Run the installed Minikube using the minikube start command. This will download the necessary images needed to execute Minikube using Docker.
- Install Kubectl in accordance with the Kubectl installation guidelines.
While setting up Kubectl, remember to add the Kubectl binary folder to your PATH environment variable. Check out this guide to set up the Kubectl binary folder and PATH correctly.
- Once the path is correctly configured, run the following command to confirm whether Kubectl is properly set:
kubectl cluster-info
Setting up the React application
First, we need to create a React app. Create a React application just as you would have done it with any React-based project.To do this, create your project folder where you want the React app to live. Change the directory to this newly created folder. Then scaffold a React application using the create-react-app and npx as follows:
npx create-react-app my-react-app
You can go ahead and check more command tags you can use (e.g., the package manager you want to use or if you want to run React with Typescript). Check out this guide for more insights.
Once the above command is executed and the React application is created, access the newly created directory:
cd my-react-app
Then run this command to test if the new app works correctly:
npm start
Test the application on the browser using the localhost URL:
http://localhost:3000/
This should serve you a basic React app. You can use the created application to progressively build any extensive application of your choice.
Write the Dockerfile
A Dockerfile sets the instructions on how to run an application on Docker. A Docker command will access the instructions written on a Dockerfile and spin up a Docker image to host the application on Docker.
The application we’ve built is currently running locally. To ship the application to Docker, we need to make sure that every file the app needs to run and the commands to execute the application are within Docker.
To do this, create a Dockerfile file at the root directory of your React application. Then write the Docker instructions as follows:
- Set the application base image. Here, React runs on Node.js. Therefore, we need a Node.js image from the Docker hub. This is the application image from which the application runs on, just as it would locally:
# set the base image to build from
FROM node:alpine
Docker supports different Node.js images with different tags and versions. Check Docker Hub to get different Node.js Image Variants for your Dockerfile.
- Create a working directory. This is the working folder where the app will run inside the container:
# set the working directory
WORKDIR /app
- Copy the dependencies files to the app directory
# copy package files
COPY package.json ./
COPY package-lock.json ./
Run the install command to install the dependencies to the Docker app directory:
# install dependencies
RUN npm install
Copy all the project files to the Docker app directory:
# copy everything to /app directory
COPY ./ ./
Add a command to run the application inside the Docker container:
# run the app
CMD ["npm", "start"]
Also, you can add a .dockerignore
file. This allows you to add files and folders you don’t want to copy to Docker, such as the node_modules
folder. Add them to the .dockerignore
file.
node_modules
.git
npm-debug.log
Dockerfile
Running the React app using Nginx
Alternatively, you can run the React app using Nginx. Nginx allows you to serve web applications using Docker. It also creates light images that are smaller in size. This runs optimized lightweight images to help reduce the size of your production builds.
Since we are creating a deployable image, I would recommend using the Nginx server to run the Dockerfile script.
If you want to work with a ready-for-production image, you need to build a production codebase. To do that, run the following command inside the React directory:
npm run build
Next, use Nginx to serve your application with the following Dockerfile:
FROM nginx:alpine
COPY build/ /usr/share/nginx/html
Running the React app on Docker
Once the installation is ready, build a Docker image for this application using the following command:
docker build -t <your-image-name> < Dockerfile filepath>
In this case, the command will be:
docker build -t react-app .
This will create an image in Docker based on the Dockerfile. The process may take some time based on your internet speed. Once complete, the created image will be available in your Docker instance.
To test if this image works correctly inside Docker, run a container to execute the image as described using the following command:
docker run -it -p <Your app Docker image port>:<Your app container Host port> <Your created-docker-image-name>
The final command should be:
docker run -it -p 4000:3000 react-app
This will build a container that runs this application. You can test the application using the local URL of your app container host port, which is 4000
in this case.
Open https://localhost:4000
in a browser and you will be served with a Dockerized React app.
Let’s dive in and run the created image using Kubernetes. There are two ways to build your Docker images in Kubernetes. These are:
1. Using a Docker Hub-deployed image.
To use this option, you need to push your image to the Docker registry.
To push your image, log in to your Docker registry. (Create a new account if you haven’t set one.) Then, run the following command:
docker login
Provide your Docker Hub username and password. If this process was successful, a Login Succeeded
message will appear on your terminal.
Next, build the image using your Docker Hub username as demonstrated in the following Docker push command:
docker build -t <docker-hub-username>/react-app
Here, ensure you set your Docker Hub username. For example:
docker build -t kimafro/react-app .
Finally, push the image to Docker Hub:
docker push <docker-hub-username>/react-app:latest
For example:
docker push kimafro/react-app:latest
2. Using a locally built Docker image.
Here, you instruct Kubernetes to pull the image from the local machine.
This guide will use an image deployed to the Docker Registry. To build your Kubernetes using the local image, check this guide and learn the right steps to run local Docker images in Kubernetes.
Deploying the Docker image to Kubernetes
The Docker image is ready, and it’s working as expected. Let’s deploy it to a Kubernetes cluster. First, create a Kubernetes deployment file. On your React app root directory, create a deployment.yaml
and add the following configurations:
apiVersion: apps/v1
kind: Deployment
metadata:
name: react-app
Set up the number of pods to run and the access to the Docker image and container:
spec:
replicas: 2
selector:
matchLabels:
app: react-app
template:
metadata:
labels:
app: react-app
spec:
containers:
- name: react-app
image: kimafro/react-app:latest
imagePullPolicy: Always
ports:
- containerPort: 80
---
Note that the name is the name of the image we created earlier. The image name should start with your Docker Hub username where you pushed the image. Make sure you copy the name to this file accordingly.
Next, add the Deployment service configurations:
apiVersion: v1
kind: Service
metadata:
name: react-app
spec:
type: NodePort
selector:
app: react-app
ports:
- port: 80
protocol: TCP
targetPort: 80
nodePort: 31000
Now, go ahead and open a command line and run the following to ensure the Minikube is running:
minikube start
Then create a deployment using the kubectl command:
kubectl apply -f deployment.yaml
Once done, check that the deployed app is running on the set deployment pod:
kubectl get pods
Next, confirm the deployed service is up and running:
kubectl get service
Note: Before proceeding, ensure the pods are running. The above image shows kubectl creating the container to access the deployed image. You can rerun the kubectl get pods
command to confirm the pods are running correctly:
react-app-6bccbdb7d-ct9wc 1/1 Running 0 91s
react-app-6bccbdb7d-kbtm5 1/1 Running 0 6m
Alternatively, you can run the following Minikube command to get a UI that will let you manage your deployments, services, and pods:
minikube dashboard
Finally, access the Kubernetes-deployed application service:
minikube service react-app
This will assign Kubernetes a static local URL and run your application on the browser. If the application is not automatically served on your browser, use the above-logged URL to access the application. For example, the above application will be served on https://127.0.0.1:2376
. At this point, you should be able to access the same application we built locally with Docker using the created Kubernetes cluster.
Learn more about building React apps with Kubernetes
I hope you found this helpful! To learn more about building modern applications with Kubernetes, check out the rest of our Kubernetes articles.
This blog post was created as part of the Mattermost Community Writing Program and is published under the CC BY-NC-SA 4.0 license. To learn more about the Mattermost Community Writing Program, check this out.