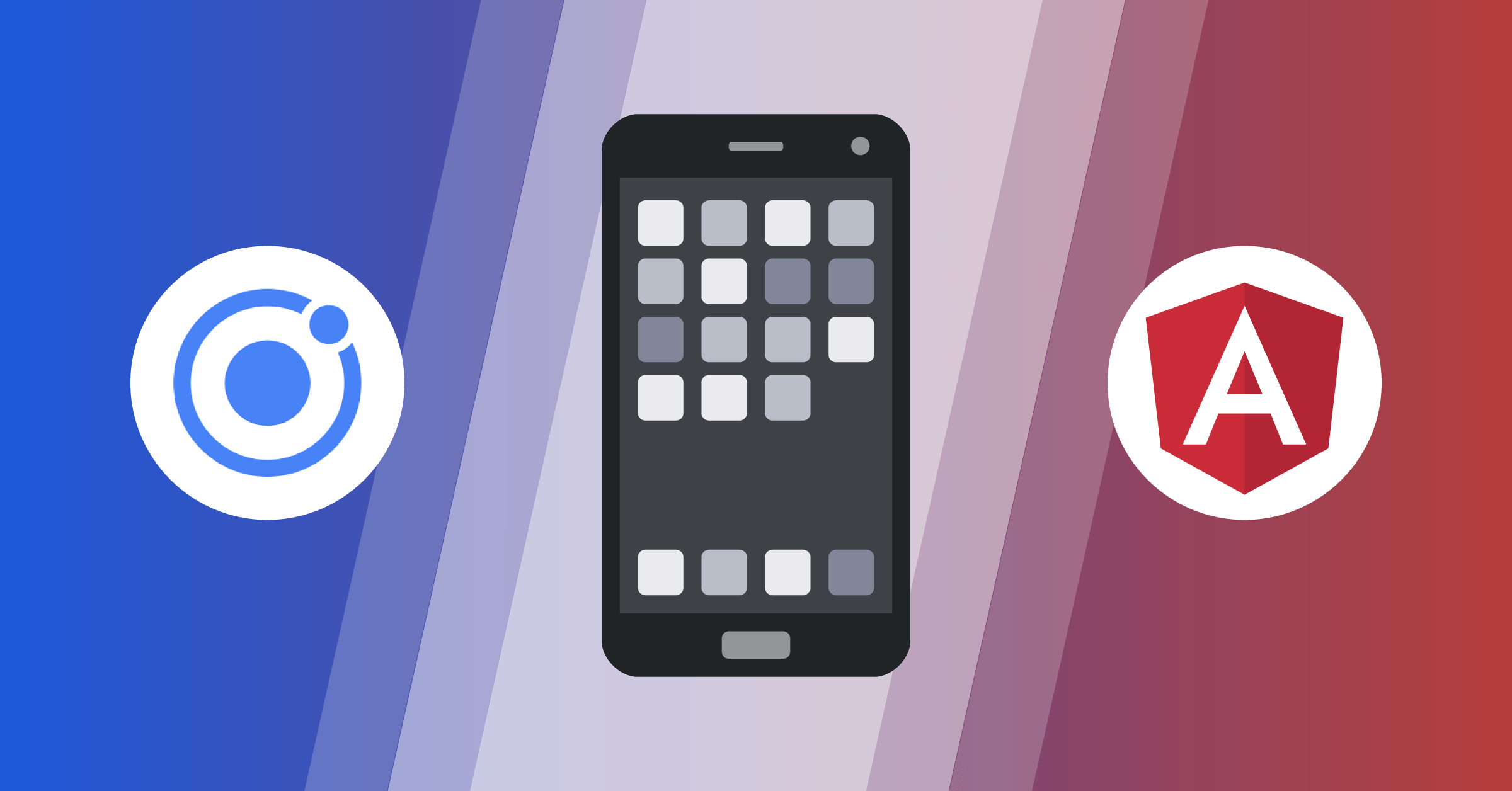
Cross-Platform Mobile Apps with Ionic & Angular: Tabs
Ionic is a popular mobile development framework that allows developers to use web technologies to produce mobile applications that work on iOS and Android. Roughly 20% of applications available on Google Play are built with Ionic, which has been a powerful mobile development framework for nearly 10 years.
Ionic allows developers to create mobile apps with a few ready-made templates; one of the more popular templates is the tabs template. This post will showcase how Ionic and the Angular framework can be used to create a mobile application with the tabs template.
Installing the Ionic CLI
The first step required to work with Ionic is to install the Ionic CLI globally via node and npm. Before you embark on this step, you must ensure that your computer has NodeJS runtime installed. For this tutorial, we will use the Ionic CLI via npx. That way, we’re always using the latest version.
Creating the Ionic tab application
In a terminal window, run the following command to create a new Ionic application based on the tabs template using the Angular framework:
npx @ionic/cli start myApp tabs --type=angular
Now that the Ionic application is created, you need to ensure that you switch directories in the myApp
folder (cd myApp) before you can go ahead and run the application. Installation will ask if you want to make a free Ionic account, but it’s not necessary. Typically, you would run the following command to test an Ionic application in the browser:
npx @ionic/cli serve
The ionic serve command will open up a browser window with your Ionic application running on localhost:8100
. However, for the purposes of this tutorial, you should run your app using a different command:
npx @ionic/cli lab
The ionic lab
command allows you to have a multi-view of your application running on iOS and Android simultaneously. If you’ve already served your application, ensure that you kill that server instance in the terminal first (using CTRL + C) before you serve your application using the ionic lab
command.
Note: Your terminal will likely ask you to install the ionic lab package when you run the ionic lab command. Be sure to select yes and allow the installation of the necessary packages to run the ionic lab command. If everything goes as planned, your application will be served at localhost:8200
, and you will see a screen that will closely remember the image below:
Exploring the Ionic project
The preview of your application shows that even though we only have one codebase, our Ionic app has two distinct looks based on IOS & Android. The designs, font type, and icons are just a few distinct, noticeable differences that Ionic magically provides.
If you look at the application’s structure, you will notice that the myApp application is not different from a typical Angular application. The Ionic CLI uses the Angular CLI behind the scenes to create your application, but it integrates to bridge that framework with the Ionic application. The first place you should explore is the tabs
folder found in the src/app
folder.
Let’s check out the tabs.page.html
file, which contains the structure that allows the tabs to operate in your Ionic application.
The <ion-tabs>
component is the crucial component that enables tabs to work in an Ionic application and allows you to add multiple <ion-tab-bar>
components, providing in-app navigation. Each <ion-tab-bar>
component allows you to have an <ion-tab-button>
within it, and you can specify an icon and name using the <ion-icon>
& <ion-label>
components respectively. To have a full view of all the icons natively available to Ionic, visit www.ionicons.com to see the full list of icons available for use with Ionic natively.
Pay close attention to the tab property of the <ion-tab-button>
component, since this property corresponds with the Angular route that a tab will display by default. Feel free to change the name of the tabs by editing the values found within the <ion-label>
. While you’re at it, play around with the icons to see if you can change them to something of your liking.
If you look at the tabs-routing.module.ts
file in the tabs folder, you’ll see a self-explanatory layout of the Angular routes created within this file where the routes tab1
, tab2
& tab3
are all declared.
Persistent tab navigation state
When working with tabs in Ionic, one prominent key feature is the built-in state across tabs. For example, if you are on tab1, navigate to a different page within tab1, select tab2, and come back to tab1, the navigation state remains intact in a cached state, even maintaining the scroll position of the page.
We need to generate a new Ionic page to test this feature. We will call this page Test
. To generate this page, we will use the Ionic CLI. In a terminal window with the myApp directory being the active one, run the following command
npx @ionic/cli generate page Test
The command above tells the Ionic CLI to generate a new page called test. If you look at your app folder structure, you’ll find a new folder called test with some files. Another file you should look at is the app-routing.module.ts
file. Within it, you’ll find a new route called test
that the Ionic CLI automatically added. This route is now a high-level route, but to achieve our goal, you will need to make it a child route of tab1 to make it accessible as a child route. To do this, you first need to open the tab1-routing.module.ts
file and add a new route for the test child component using the following code:
{
path: 'test',
loadChildren: () => import('../test/test.module').then(module=> module.TestPageModule),
}
The final result should look like this:
To finish up, you must create a link within the tab1 page that will trigger navigation to the test page. Head to the tab1 page and add an Ionic button that will navigate to the test page. To achieve this, copy and paste the code snippet below after ion-header
:
<ion-button routerLink="test">Click Here To Go To Test page</ion-button>
Ensure to add this code snippet within the <ion-content>
component for correct implementation. After doing this, you’ll see a new page with the header test
pop up when you click the button on the home page.
Now, if you click any other tab and return to tab1, you’ll see that the test page remains on screen in the same state you initially left. There’s no implementation for the back button at this point, but you can read about how to add this on the test page in the docs.
Summary
The Ionic tab-based template is only one of many templates that Ionic provides developers. It is easily one of the most popular, and we have only covered a tiny part of the capabilities that the Ionic tab can accomplish.
To learn more about this, spend some time at the Ionic component documentation, where you can find even more components made available by Ionic. Interested in reading more content like this? Browse the Mattermost Library and continue your learning.