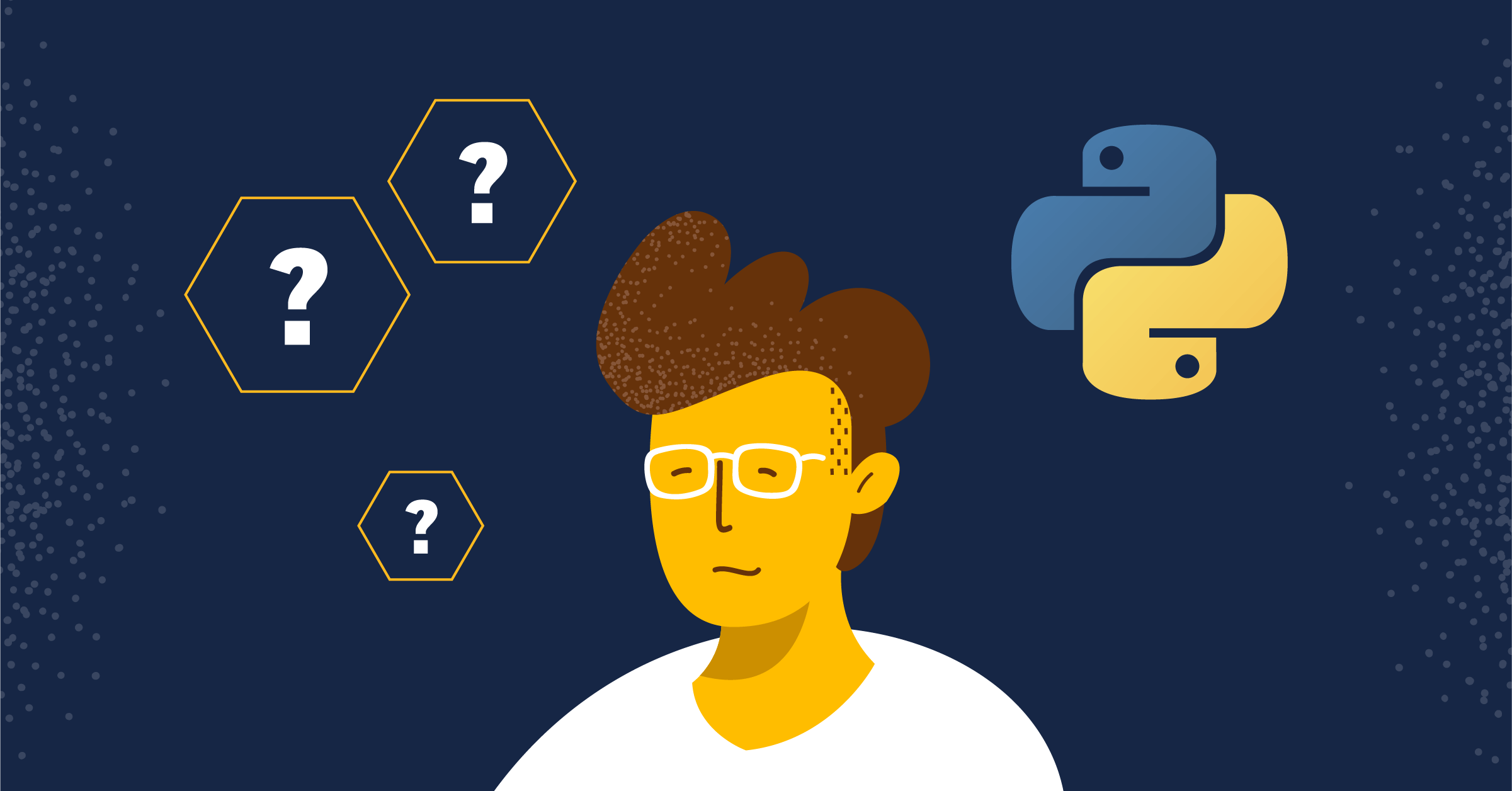
Flask Versus Django: Which Python Framework Is Right for You?
Whether you’re a new developer or a seasoned professional looking for a new Python web framework, Django and Flask offer two compelling open source options to choose from. So, how do you choose which framework is best for you? This article is designed to make that decision easier by providing an in-depth comparison of the two frameworks.
Django is a comprehensive framework for building large and dynamic web applications. It has a built-in database (ORM) and a variety of pre-built features to choose from. Though it can sometimes be complicated to get started with, Django is a good choice for rapid development and complex applications.
Alternatively, Flask is a lightweight microframework for simple website building. It lacks a built-in database but offers the ability to add other plugins for complete control and customization. It’s well-suited for those who like to build from scratch and dive deeper into the specifics.
Django is the framework of choice at companies like Google, YouTube, Instagram, Pinterest, NASA, Spotify, and Dropbox. Flask, meanwhile, is used at Airbnb, Netflix, Samsung, and RedHat.
The Major Differences between Flask and Django
Before diving into comparing some small sample applications for each framework, let’s briefly go over the major features of Django and Flask.
Key Features in Django
Django uses a Model-Template-View pattern and its packages are reusable apps, websites, tools, and other resources. It’s richly featured out of the box and supports functionality for user authentication, content administration, site mapping, RSS feeds, and other web development tasks. It’s also SEO-optimized, as sites are maintained using URLs. Additionally, it’s highly secure, providing XSS protection, CSRF protection, SQL injection protection, clickjacking prevention, SSL/HTTPS, host header validation, and other security policies.
Django is more suited for complex applications than Flask, and as such is a more complex framework. Django has a steep learning curve, but its versatility makes it very popular. It’s used for everything from CMS and social networking platforms to scientific computing platforms.
Key Features in Flask
Flask is a microframework that’s simple but extensible. It offers integrated unit testing, using the Jinja templating engine and Werkzeug WSGI toolkit. Flask supports secure cookies and has templating, routing, error handling, and a debugger. The framework focuses on initial ease of deployment but allows a huge range of Python plugins and libraries to accommodate deep flexibility and customization.
Sample Code Comparison
Let’s compare how simple it is to create a basic app using Django and Flask. This app will accept a username as the input and then display a message on the screen.
Django Sample Project
First, create a project directory. Then create a folder inside it called venv
.
On a Windows machine, use the following commands:
> mkdir djangoproject
> cd djangoproject
> py -3 -m venv venv
On a MacOS/Linux machine, use the following commands:
$ mkdir djangoproject
$ cd djangoproject
$ python3 -m venv venv
Then, activate the environment.
On Windows:
> venv\Scripts\activate
On MacOS/Linux:
$ . venv/bin/activate
Upgrade pip by using the following command:
python -m pip install --upgrade pip
Then, install Django.
python -m pip install django
To set up and start the Django project, use the following command:
django-admin startproject django_project
Django projects are made up of many site-level configuration files, as well as one or more “apps” that you publish to a web host to form a completely online application. You’ll create your app later down below.
At this point, the project directory structure should look like this:
Next, create an empty development database:
python manage.py migrate
Run the database to verify that everything works correctly.
python manage.py runserver
Now, create a Django app called myapp
with the following command:
python manage.py startapp myapp
Your directory structure should look like this:
There are a few adjustments to make before you can get started.
First, add your app name to INSTALLED_APPS
in /django_project/settings.py
.
INSTALLED_APPS = [ ..
'myapp',
]
Django uses templates as an easy way to generate HTML dynamically. Templates include both static and dynamic content components, as well as a specific syntax for inserting dynamic information. To use templates, make the following changes to DIRS
within TEMPLATES
in the same file:
import os
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(BASE_DIR, 'template')],
..}]
The URL dispatcher uses a path to route URLs to the proper view functions within a Django application. In django_project/urls.py
, add the path for myapp
project to urlpatterns
with the following code:
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('', include("myapp.urls")),
path('admin/', admin.site.urls),
]
Add the paths for the home and welcome views to myapp/urls.py
:
from django.urls import path
from myapp import views
urlpatterns = [
path("", views.home, name="home"),
path("welcome", views.welcome, name="welcome")
]
Then, add the following code to myapp/views.py
:
from django.shortcuts import render, HttpResponse
# Create your views here.
def home(request):
return render(request, 'index.html')
def welcome(request):
username = request.GET["name"]
return render(request, 'welcome.html', { 'name': username})
This code defines home to render the index.html
template and defines welcome
to get user input and render welcome.html
to show the result message.
Next, create your index.html
file and add the following code for the Welcome page to accept user input:
<!doctype html>
<html>
<title>Welcome Page - Django</title>
<body>
<p> Enter your name</p>
<form action = "welcome">
Name: <input type = "text" name="name">
<button type="submit">Enter</button>
</form>
</body>
</html>
Then, create a welcome.html
file to display the result:
Welcome {{name}}!
Run the project.
python manage.py runserver
Then, open a browser to http://127.0.0.1:8000/
. You should see a page that renders a prompt to enter your name that looks similar to this:
Entering your name and clicking the Enter button should give you the following output:
Flask Sample Project
Now that you understand the basics, let’s turn our attention to a sample project.
First, create a project directory. Then, either use the virtual environment from the Django sample, or create a new one. Next, activate the environment and install Flask using the following command:
pip install flask
Create a new Python file called app.py
.
In app.py
, add the following code to import Flask and to create a route for the home and welcome pages:
from flask import Flask, redirect, url_for, render_template, request
app = Flask(__name__)
@app.route("/")
def home():
return render_template ("index.html")
@app.route("/welcome", methods=["POST", "GET"])
def welcome():
if request.method == "POST":
name = request.form["name"]
return f" Welcome {name}!
"
else:
return render_template ("index.html")
if __name__ == "__main__":
app.run(debug=True)
This code defines home to render index.html
, and welcome to get user input from the form and display it on welcome.html
.
In the same directory as app.py
, create a folder called templates
. In it, create an index.html
file and add the following code to accept user input via form:
<!doctype html>
<html>
<title>Welcome Page</title>
<body>
<p> Enter your name </p>
<form action="#" method="post">
<p>Name:</p>
<p><input type="text" name="name" /></p>
<p><input type="submit" value="Enter"/></p>
</form>
</body>
</html>
Run the project.
python -m flask run
Then, open a browser to http://127.0.0.1:5000/welcome
. You should see a page that looks similar to the one rendered by our Django project:
Entering your name should give you the following output:
Our sample projects make one thing evident: Flask is relatively straightforward to set up and get started, but Django needs a few more steps because it’s designed for large projects with more varied capabilities.
Key Differences for Developers
Let’s examine and compare Django against Flask based on some major factors affecting developers working in the frameworks. In this section, we’ll go over each framework’s tech stack, ease of development and startup complexity, their overall capabilities, performance, supporting libraries and integrations, and finally their level of documentation and community support.
Tech Stack
Architecture
Django has a variation on the classic MVC pattern known as MVT (Model View Template) where the standard Controller is called the “View,” and the View is called the “Template.”
Flask is more open-ended, lacking a set architecture. It can make use of extensions and other configurable options and is sometimes referred to as having a DIY architecture.
Templates
Django has back ends for its own template system, dubbed the Django template language (DTL). For example:
- Variables: My first name is
{{ first_name }}
. My last name is{{ last_name }}
- Tags taking arguments:
{% cycle 'odd' 'even' %}
Flask uses the Jinja template library to render templates. It utilizes files containing both static data and placeholders for dynamic data. For example:
- Variables:
{{ foo.bar }}
or{{ foo['bar'] }}
- To use Python commands:
{% if loop.index is divisibleby 3 %}
{% if loop.index is divisibleby 3 %}
Python commands
Data Modeling
Django includes its own built-in object relational mapper (ORM) that may be used for data manipulation, querying, filtering, selection, and other standard operations.
Flask doesn’t have its own database, but it connects to other Python modules in the Python package index, such as SQL Alchemy and MongoEngine.
Ease of Development and Overall Startup Complexity
After installation and project setup, Django has built-in files and configurations. This requires more work from the developer to get started.
Flask is easier to install and use because you can use just a single command to get started. The following image illustrates the steps involved in setting up a simple Hello World program between Django and Flask:
Flask requires only a simple installation followed by the creation of an app.py file to get started. Django, on the other hand, requires you to initiate the Django project first and then build your app and configure other modifications. Django requires all of this to manage the large projects it’s intended to be used for.
Overall Capabilities
Beginners often find Django a little intimidating to get started with; many find Flask to be an easy alternative. But, although Flask can be used for multiple pages and large projects, it is not an ideal option for complex projects with databases.
Users and Admin
Many websites need user accounts managed by an admin interface. Django has a built-in user and admin framework that is easy to use. Flask, on the other hand, requires multiple extensions to achieve this functionality but offers extensions like Flask-Security that bring it up to the same level of ease as Django. Comparing the frameworks in this area comes down to ease of use versus customization.
Django users can use the built-in django.contrib.auth
user model. Administration is a highly customizable permission system, and it’s also easy to use.
Flask doesn’t have built-in users but can use the popular Flask-Login extension to achieve the same effect. Administration in Flask uses Flask-Admin, is highly customizable, and can work with SQLAlchamy, MongoEngine, and others.
For argument’s sake, let’s say everyone is using the same set of extensions for projects like flask-admin to create an admin panel. In such a scenario, you open possibilities for security issues with Flask. This is because you can’t customize it to your needs. You can, however, use Flask-Security to help take care of these concerns.
Apps in Django vs Blueprints in Flask
Django developers use apps, whereas Flask developers use blueprints to create components. They both serve the same role of splitting the code into independent modules to provide reusability. Django apps are more extensive and numerous, but they’re also more difficult to work with. Flask blueprints are simpler and easier to integrate into a project.
API Comparison
APIs are becoming more widespread for web applications, and they frequently demand different patterns than HTML web pages.
The Django REST framework includes multi-layered abstraction, authentication controls, serializers, and extensive documentation. It’s important to note that the Django web framework only works easily with relational databases.
Flask offers multiple extensions that work together with the Marshmallow framework-agnostic library. So, it can provide more flexibility than Django. But you have to configure it yourself.
Performance
Flask is faster than Django, although the difference might be too small to notice in many cases. By being a lightweight framework implemented mostly on small projects, it has better performance than large frameworks like Django. Choosing which framework to use mostly depends on your project size and requirements.
Supporting Libraries/Plugins and Extensions
Both Django and Flask provide the ability to integrate with other libraries or extensions to enhance their performance. Let’s look at some examples.
For Flask:
- Flask-AppBuilder is a web application generator that utilizes Flask to generate code for database-driven applications depending on user-specified parameters.
- Flask-Authorize is a Flask plugin that makes it easier to implement access control lists (ACLs) and role-based access control (RBAC) in web applications.
- Flask DebugToolbar is a Flask port of the well-known Django Debug Toolbar project.
- Flask RESTX is a Python extension for easily including RESTful APIs in your applications.
For Django:
- Django Extensions includes shell_plus, which will automatically import all models in your project. It includes several important commands, such as
admin_generator
,RunServerPlus
, andvalidate_templates
. - Django-allauth provides authentication, registration, and account management solutions.
- Django Compressor consolidates all of your JS and CSS files into a single cached file. It also has Jinja templating and ReactJS support.
- The Celery application, which handles long-running, data-intensive tasks that can’t be handled in the request-response cycle, now supports Django out of the box.
Community Support and Documentation
Django’s total documentation, with its exceptionally detailed guides and tutorials, is more thorough than Flask’s. Django also has a larger community, and therefore, more support. Django GitHub has 63.1k stars, whereas Flask has 58.4k stars (as of March 2022). This illustrates that more people have shown interest in Django than Flask based on their project requirements. Additionally, Stackoverflow currently has over 285,000 questions tagged [django]
, but only just over 49,000 questions tagged [flask]
.
Side-by-Side Comparison
Both Django and Flask are well suited for their specific, differing roles. Let’s briefly examine the strengths and weaknesses of both frameworks again.
Pros and Cons of Django
Pros:
- Django is simple to install and use with many in-built features.
- It has an easy-to-use interface for administrative tasks.
- You can use Django for end-to-end application testing.
- The REST Framework provides extensive support for a variety of authentication mechanisms.
- It ensures speedy development with a robust built-in template design.
- Django’s caching framework includes a wide variety of cache techniques.
- It has a comprehensive set of tools.
- Django’s large community offers great support and documentation.
Cons:
- Django’s monolithic style can make things too difficult and concepts too fixed.
- Prior understanding of the framework is needed to accomplish many things.
- For a simple project, there are too many functions in Django’s high-end framework.
- URL dispatching using controller regex complicates the codebase, which is heavily reliant on Django ORM.
- Django has less flexible design considerations and fewer components.
- It has a steep learning curve.
- Its WSGI service can handle only one request.
- You’ll need some familiarity with regex for routing.
- Internal subcomponents are tightly coupled.
- Components can be deployed together. This might lead to confusion between developers if, for example, you create and use too many template components.
Pros and Cons of Flask
Pros:
- As an independent framework, it allows for architectural and library innovation.
- Flask works well for small projects.
- It ensures scalability for simple applications.
- It’s easy to quickly create prototypes.
- Routing URL functions using the Werkzeug WSGI library is simple.
- Application development and maintenance are painless.
- Flask is adaptable and well-suited for emerging technologies.
Cons:
- In most circumstances, the time to Minimum Viable Product (MVP) is slower.
- It has an inadequate database and ORM options out of the box.
- Flask is not appropriate for large applications or projects.
- It lacks built-in admin functionality for managing models and for inserting, updating, or deleting records.
- Complicated systems have higher maintenance expenses.
- Maintenance is more difficult for bigger deployments.
- Flask suffers from a smaller community, hampering support and slowing its growth.
- The framework is not standardized, so the developer of one project might not easily understand another project.
- There are fewer tools available, so developers often need to build tools from scratch, which is time-consuming.
Final Thoughts on Choosing Flask vs. Django
Choosing which framework to work with is entirely up to you, and will depend on the size and scope of your project. As you saw throughout this article, Flask is better suited for basic, single-page web apps with minimal or no database requirements, though it may produce high-performance web apps if you’re careful to keep them small. Flask’s ability to use Python extensions and libraries provides versatility, but it also introduces extra work into the process. The platform is best suited for developers who want to build things from the ground up and dive deeper into the specifications.
Django is an excellent solution for more highly complicated web apps that rely on databases. Because of its many specifications out of the box, it’s simple for someone with no web programming experience to get started and construct an app using built-in templates and Django’s other features. It also enjoys an active community that provides thorough support and documentation.
This article has highlighted the differences between Django and Flask and should help you determine which of these frameworks will best benefit the development of your next app. Looking to learn more? To dive deeper and find other articles like this one, check out Mattermost and visit the Mattermost Blog.
This blog post was created as part of the Mattermost Community Writing Program and is published under the CC BY-NC-SA 4.0 license. To learn more about the Mattermost Community Writing Program, check this out.