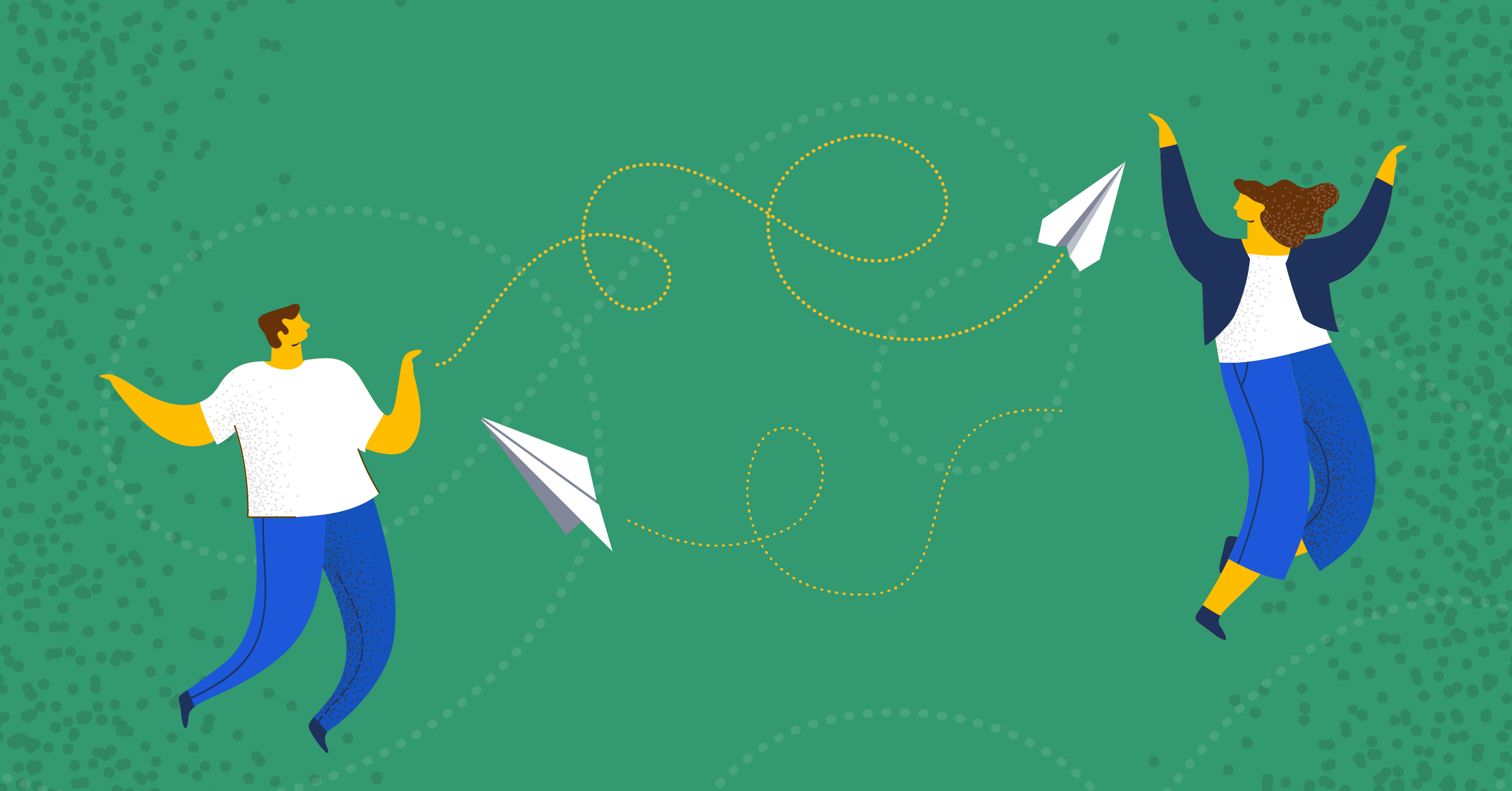
Mattermost integrations: Sending and receiving data with the Mattermost API
The Mattermost API is the most powerful way to facilitate interactions between Mattermost and external systems
(Originally published at controlaltdeliet.be)
In the first three installments in this series, you learned how to send and request data with outgoing webhooks, incoming webhooks, and slash commands. In this article, you learn how to do this with the Mattermost API.
Setting up webhooks or slash commands in Mattermost is very easy. Using the Mattermost API requires a few more steps. At the same time, the API is way more versatile and powerful than the other methods. Once you have made your first connection to the API, you will love it!
Create a Personal Access Token
You can work without a Personal Access Token and login with a username and password. If you go that route, you’ll receive a temporary access token that will expire.
Personal Access Tokens, on the other hand, do not expire. They are also more secure because you don’t have to store username and password in your code. Further, using a personal access token gives you also full access to the API.
Follow these steps to create a Personal Access Token.
1. Go to the Menu
2. Go to Account Settings
3. Go to Security and click Edit to manage your Access Tokens
4. Create your Personal Access Token in the next screen and give it a description
5. If you are generating an Personal Access Token with sysadmin rights, you’ll receive a warning.
6. Your token is created. Make a copy of it because you won’t be able to see it again.
7. Forget to copy your token? You’ll have one more chance.
Write some code
By this point, you’ve done everything you needed to do to gain access to the Mattermost API.
Now, it’s time to learn how to use the API in Python.
We’ll be using the Mattermost Driver, and there are also drivers available for JavaScript, Go, and PHP.
1. First things first: Install the dependencies
pip install mattermostdriver
2. Now, let’s build some magical Python code
First, we import the necessary classes:
from mattermostdriver import Driver
import json
3. Next, we connect to Mattermost
from mattermostdriver import Driver
import json
mm = Driver({
'url': 'your.mattermost.server.fqdn',
"token":"s1ern7edy3do9ggg9zdhakzyaw", ## THE TOKEN THAT YOU JUST CREATED
'scheme': 'https',
'port': 443
})
mm.login()
4. Now, let’s write something to a channel!
You have to fill in two variables. Your teamname and the channel name. If you have spaces or special characters in your channel name, you can find the channel name by going to the channel in your browsers. You’ll see the channel name in the URL.
from mattermostdriver import Driver
import json
channel_name="YOUR-CHANNEL-NAME"
team="YOUR-TEAMNAME"
mm = Driver({
'url': 'your.mattermost.server.fqdn',
"token":"THE TOKEN THAT YOU JUST CREATED",
'scheme': 'https',
'port': 443
})
mm.login()
channel=mm.channels.get_channel_by_name_and_team_name(team, channel_name)
channel_id=channel['id']
mm.posts.create_post(options={
'channel_id': channel_id,
'message': 'This is a test through the API'
})
And here’s the output:
5. Listening to events
In order to listen to Mattermost, you need to connect to a websocket. The events are passed to an event listener. In the example provided, you will print all the events that are occurring. You receive the events in JSON.
from mattermostdriver import Driver
import json
mm = Driver({
'url': 'your.mattermost.server.fqdn',
"token":"THE TOKEN THAT YOU JUST CREATED",
'scheme': 'https',
'port': 443
})
mm.login()
async def my_event_handler(e):
message=json.loads(e)
print(message)
mm.init_websocket(my_event_handler)
More information on the Mattermost API
You can learn a lot by watching the output that the event handler is printing.
Mattermost provides great information on the Mattermost API. Take a look at that link, and you’ll see you can do almost anything through the API.
The Python driver is well-documented, too! You can read all about it here.